Garbage collectors
Reference counting garbage collectors
Pros
- Easy to implement
Cons
- Additional data (field) needed to store reference counter
- Atomicity of counter changing? -> very slow
- If counter change is not atomic -> GIL needed instead
Generational garbage collectors
- Based on assumption that objects usually die young
- Used in Python VM and in JVM as well
- Heap is splitted into so called generations
- 3 generations in Python VM
- 2 generations in JVM
Pros
- Allocation is for free
- Deallocation can be started in its own thread or threads
Cons
- Hard to implement (correctly)
- Stop-the-world full GC is needed sometimes
Java heap memory model
Generations
- Two generations - young and old
- Objects are created mainly in young generation
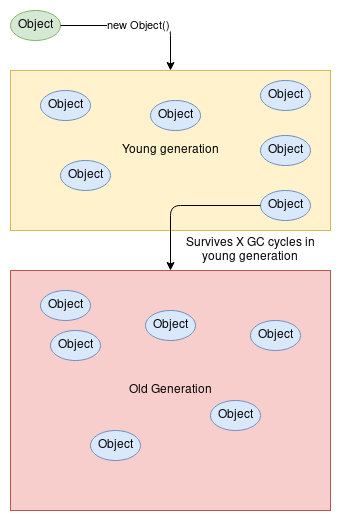
Young generation structure
- Eden space for newly created objects
- Two survivor spaces
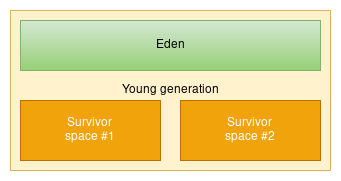
Objects are created in eden
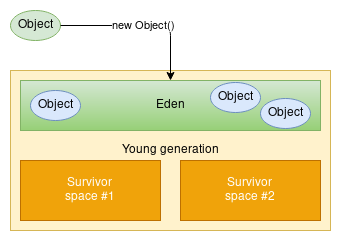
When eden is full, objects are moved into selected survivor space
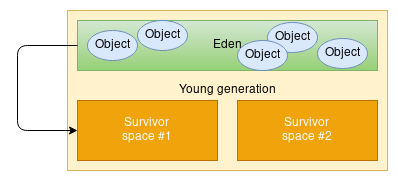
At the end of this operation, eden is free
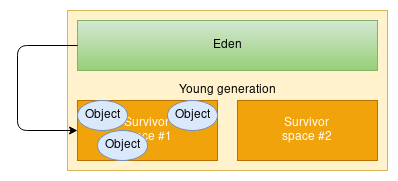
After many cycles of empying eden, the survivor space gets full:
- Regular GC starts
- Live objects are moved into second survivor space
- “Survive counter” is increased by one
- This means the space is compact
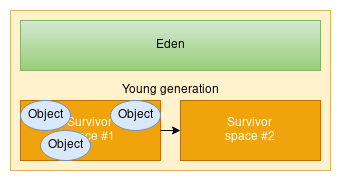
At the end of regular GC, one survivor space if free and second is compacted
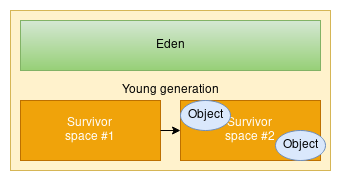
After many cycles the survivor space is going to be full:
- Object that survive many cycles of regular GC is moved into old generation area
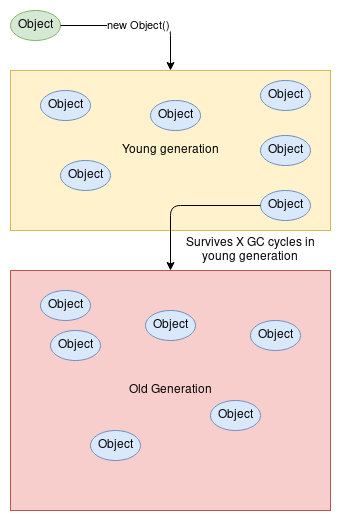
Full GC needs to be started from time to time on old area. It is slow, but usually
many object survives.
More speedup possible
- Thread Local Allocation Buffers (TLAB)
- Allocation = add a constant to offset pointing to free region
- Nothing more!